Many blog owners love to have a slider for featured posts in their blog home page these days. A slider gives draws the attention to the blog posts selected by the owner and allows the visitors to quickly glance through some of the best posts. It is a smart and welcomed way to promote the blog with its own posts. Many blog owners seek help from various plugins to achieve the slider for featured posts. But I prefer to code it directly in the theme I work on, while using the existing ‘Sticky Post’ feature in WordPress to achieve the same effect.
Disclaimer: This may not be the only nor the best way for anyone to achieve the same presentation, but I intend to share it as a reference for interested people.
There are several reasons I usually choose to use the Sticky Post feature instead of implementing another custom field or tag or category etc. First, I notice the native WordPress Sticky Post feature is not in use in most of the blogs (note: personal observation), and I think this native feature should be more well utilized.
Second, I think the current way presenting Sticky Posts in WordPress takes away too much attention from the worthy latest posts because Sticky Posts take up the place before the latest posts at the same content area. A separate area to display the Sticky Posts such as the post slider area is a good way to give attention to some ‘selected’ posts while not attracting too much attention away from the latest posts.
Third, implementing the post slider using a tag or a category is more like a hack because tags and categories are supposed to describe the contents of the blog post. Sometimes there may be a need to present contents with a certain tag or category differently (e.g. tag templates or category templates), but the main point of using tags and categories should be to describe the blog post contents, not merely as a way to differentiate the way blog posts are presented.
Enough said. Let’s get into how I do it.
Let’s say I have these blog posts and several of them are made ‘Stick Posts’:
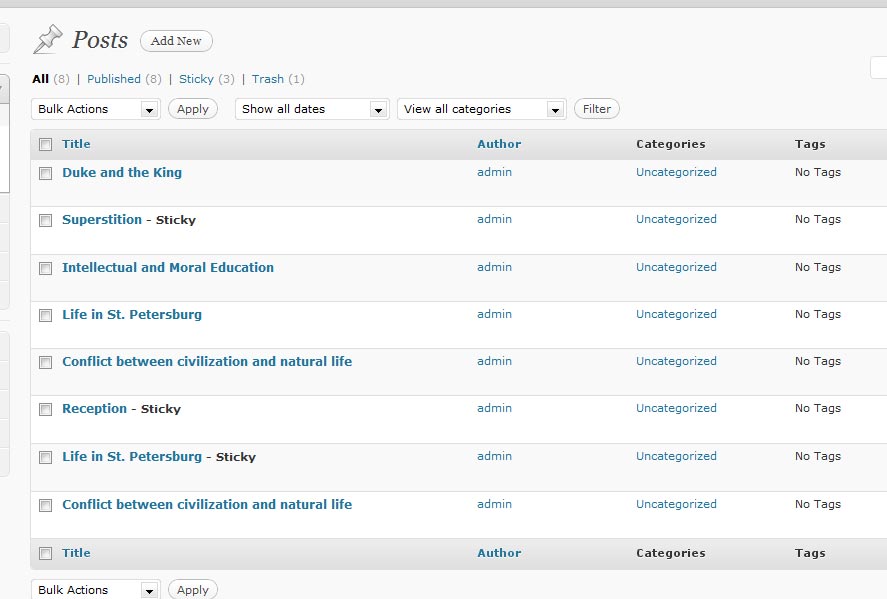
In the default Twenty Ten theme, each of the Sticky Posts appears in a box in a light-blue background with a thick black top border line in the home page of the blog, in the main blog posts area above all most recent posts:
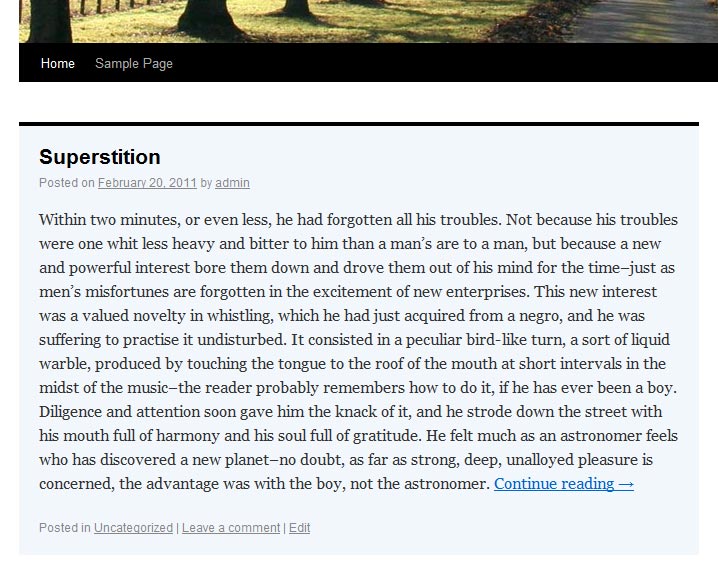
MooTools and noobSlide
Since in this post, I’m going to use noobSlide as our content slider, we need to include MooTools JavaScript library. Go to the functions.php
file in the template and add the following codes at the bottommost.
function register_custom_js() { wp_register_script( 'mootools', 'http://ajax.googleapis.com/ajax/libs/mootools/1.3.0/mootools-yui-compressed.js', '1.3.0' ); wp_register_script( 'noobslide', get_bloginfo('template_directory') . '/_class.noobSlide.packed.js' , array( 'mootools' ) ); } add_action( 'init', 'register_custom_js' ); function enqueue_noobslide() { if ( is_home() ) { // our slider only appear in the home page, so load the script only in home page wp_enqueue_script( 'noobslide' ); } } add_action( 'wp_print_scripts', 'enqueue_noobslide' );
Post Thumbnail Support
In this tutorial, I’m going to use post thumbnails in my slider. If your theme has not yet added the feature, you can add it using the following codes in your functions.php
:
function custom_theme_setup() { add_theme_support( 'post_thumbnails' ); } add_action( 'after_setup_theme', 'custom_theme_setup' );
Also make sure your Sticky Posts has set the ‘Featured Image’ (BTW, WordPress should standardize the use of name).
Custom ‘Read more’ Link
In the default Twenty Ten theme, a custom “Read more” link is used. I’m going to change it to use my own customized “Read more” link so I can place a class for styling.
I will comment out this line in the Twenty Ten functions.php
file:
//add_filter( 'get_the_excerpt', 'twentyten_custom_excerpt_more' );
Then, I will add the following to the same file:
function custom_excerpt_with_more( $excerpt ) { if ( has_excerpt() && ! is_attachment() ) { global $post; return $excerpt . '<p class="slider-more"><a href="' . get_permalink( $post->ID ) . '">Continue reading</a></p>'; } return $excerpt; } add_filter( 'get_the_excerpt', 'custom_excerpt_with_more' ); function custom_excerpt_more( $more ) { global $post; return '<p class="slider-more"><a href="' . get_permalink( $post->ID ) . '">Continue reading</a></p>'; } add_filter( 'excerpt_more', 'custom_excerpt_more' );
custom_excerpt_with_more()
function is for automatic excerpts or user-specified excerpts with read-more tag. custom_excerpt_more()
is for hand-crafted excerpts.
CSS and JavaScript
Then, we add the custom CSS and JavaScript codes in functions.php
to make the theme serve them when we are in the home page where the slider will appear.
function custom_slider_css_js() { if ( is_home() ) { // only in home page ?> <style type="text/css"> #slider{border:1px solid #ccc;width:640px;position:relative;overflow:hidden;height:213px;margin-bottom:20px;} #mask{position:absolute;height:213px;} .items{float:left;height:213px;width:640px;position:relative;} .items .wp-post-image {float:left;} .info{width:320px;float:left;font-family:Arial, Helvetica, sans-serif;} #content .info h2{font-size:20px;font-weight:bold;color:#1c3f95;margin:10px 10px 15px 10px;} #content .info p{font-size:11px;color:#1c3f95;line-height:16px;margin:10px;} .info a{font-size:10px;padding:5px 7px;background:#e1e1e1;text-decoration:none;text-transform:uppercase;font-weight:bold;color:#1c3f95;} .info a:hover{color:#ffffff;background:#1c3f95;} #slider .handle{position:absolute;bottom:0;right:5px;line-height:10px;text-align:center;font-size:25px;font-weight:bold;} .handle a{color:#ccc;height:20px;width:20px;display:inline-block;cursor:pointer;} .handle .active{color:#1c3f95;} .handle a:hover{color:#1c9f65;} </style> <script type="text/javascript"> /*<![CDATA[*/ window.addEvent('domready',function(){ var nS8 = new noobSlide({ box: $('mask'), items: $$('#mask .items'), size: 640, autoPlay: true, interval:8000, handles: $$('.handle a'), onWalk: function(currentItem,currentHandle){ $$(this.handles).removeClass('active'); $$(currentHandle).addClass('active'); } }); var handle = $$('.handle a'); handle.each(function(el,i){el.addEvent('click',nS8.walk.bind(nS8,[i,true]));}); }); /*]]>*/ </script> <?php } } add_action('wp_head', 'custom_slider_css_js' );
WordPress Theme Modification
Next, go to the theme template file that display the Loop in the home page, in this case, it is the loop.php file in the Twenty Ten theme. Place the cursor right before the Loop area and we can start putting our codes for the slider.
<?php if ( is_home() ): $sticky = get_option('sticky_posts'); $slider = new wp_query( array( 'post__in' => $sticky ) ); ?> <?php if ($slider->have_posts()): $count = 0; ?> <div id="slider"> <div id="mask"> <?php while ( $slider->have_posts() ): $slider->the_post(); $count++; ?> <div class="items"> <?php the_post_thumbnail( array( 320, 213 ) ); ?> <div class="info"> <h2><?php the_title(); ?></h2> <?php the_excerpt(); ?> </div> </div> <?php endwhile; ?> </div> <div class="handle"> <?php for ($count; $count > 0; $count--): ?> <a>•</a> <?php endfor; ?> </div> </div> <?php endif; ?> <?php endif; ?>
At this point of time, when you go to the home page of the blog, you will see the slider is up and running already and we are almost done:
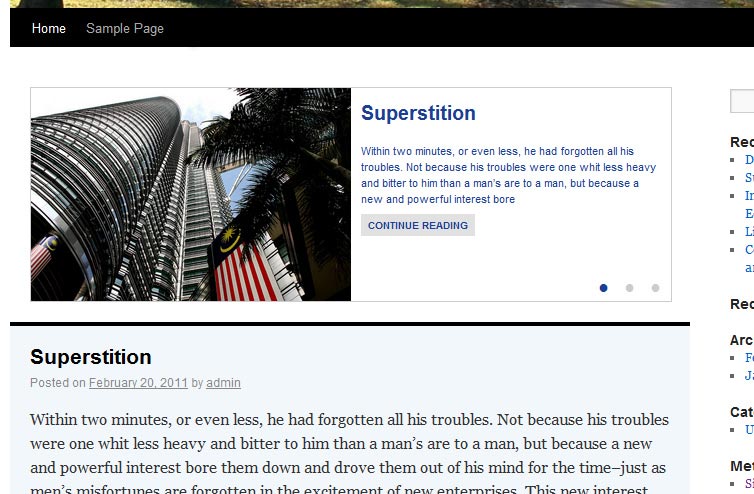
But wait, we are not done yet. Notice the main blog posts area still have the Sticky Posts? We need to get rid of them to return the attention taken away from the latest posts. To accomplish that, we will add the following codes right after our content slider codes:
<?php if ( is_home() ): $sticky = get_option('sticky_posts'); $slider = new wp_query( array( 'post__in' => $sticky ) ); ?> <?php if ($slider->have_posts()): $count = 0; ?> <div id="slider"> <div id="mask"> <?php while ( $slider->have_posts() ): $slider->the_post(); $count++; ?> <div class="items"> <?php the_post_thumbnail( array( 320, 213 ) ); ?> <div class="info"> <h2><?php the_title(); ?></h2> <?php the_excerpt(); ?> </div> </div> <?php endwhile; ?> </div> <div class="handle"> <?php for ($count; $count > 0; $count--): ?> <a>•</a> <?php endfor; ?> </div> </div> <?php endif; ?> <?php $args = array_merge( $wp_query->query, array( 'post__not_in' => $sticky ) ); query_posts( $args ); ?> <?php endif; ?>
All Done!
Now enjoy your fully functional content slider. =)
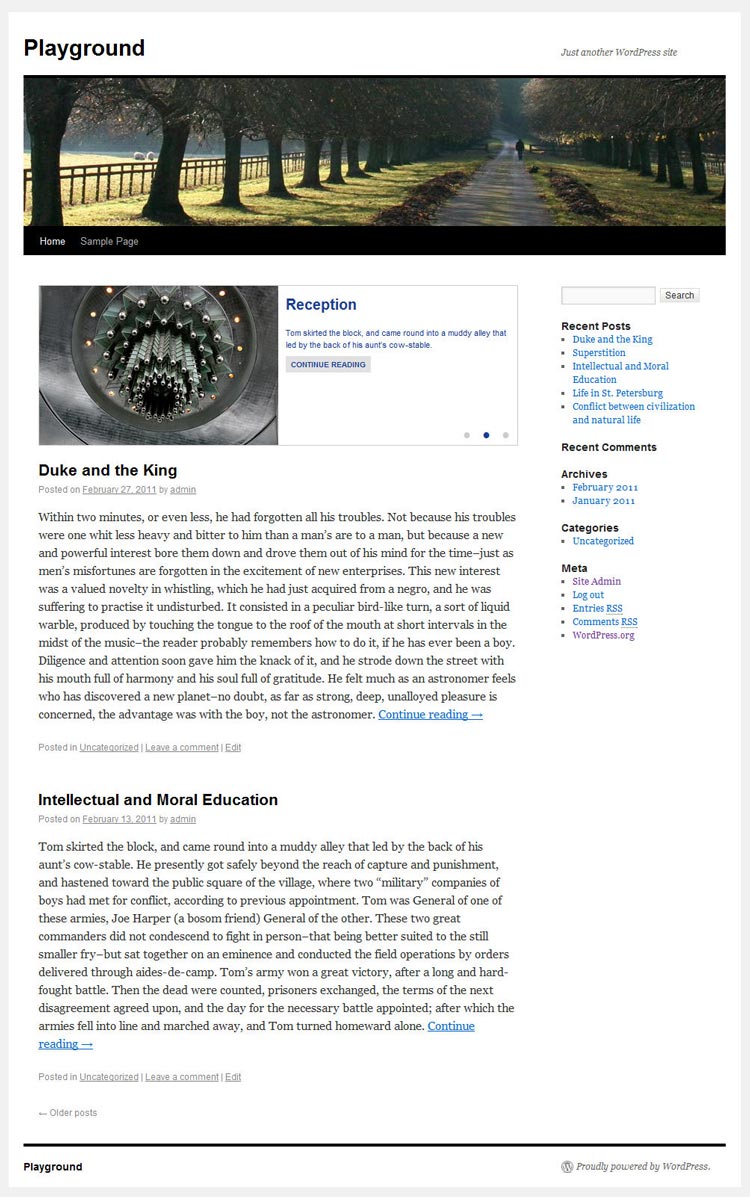
Images in the sample blog courtesy of phalinn.